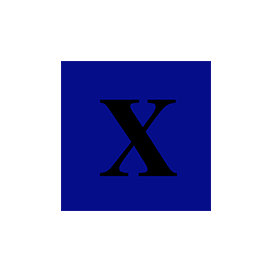
docxtemplater
Generate Word DOCX from Templates via JavaScript
Open Source JavaScript library to create, modify & convert Microsoft® Word DOCX files.
What is docxtemplater?
docxtemplater is an open source JavaScript library that helps in creating and editing Word DOCX files from a template. The Word JavaScript library enables users to customize generated documents using Word itself. The library is easy to handle and does not require any technical skills to edit a Word template. The library has also provided several modules for specific functionality.
The docxtemplater library supports several features related to DOCX file creation and handling such as adding images, inserting formatted text in a Word document, inserting headers/footers, image replacement with any existing properties, creating tables, adding watermark text, updating page margins, insert footnotes to a document and much more.
How to Install docxtemplater?
The recommend and easiest way to install docxtemplater is via npm. Please use the following command for a smooth installation.
Install docxtemplater via npm
npm install docxtemplater pizzip
Generate Word DOCX via JavaScript
The docxtemplater library helps in creating DOCX documents in a Node.js app as well as in the browser with ease. It also allows to modify the existing DOCX documents to insert tables, images, text, paragraphs, and more.
Update Word DOCX via JavaScript
const PizZip = require("pizzip");
const Docxtemplater = require("docxtemplater");
const fs = require("fs");
const path = require("path");
// Load the docx file as binary content
const content = fs.readFileSync(
path.resolve(__dirname, "input.docx"),
"binary"
);
const zip = new PizZip(content);
const doc = new Docxtemplater(zip, {
paragraphLoop: true,
linebreaks: true,
});
// Render the document (Replace {first_name} by John, {last_name} by Doe, ...)
doc.render({
first_name: "John",
last_name: "Doe",
phone: "0652455478",
description: "New Website",
});
const buf = doc.getZip().generate({
type: "nodebuffer",
compression: "DEFLATE",
});
// buf is a nodejs Buffer, you can either write it to a
// file or res.send it with express for example.
fs.writeFileSync(path.resolve(__dirname, "output.docx"), buf);
Insert & Manage Tables in Word Documents
The docxtemplater library enables software developers to create a table with just a couple of lines of JavaScript code. The library has included several methods for creating and managing tables in a document such as tables creation from the scratch, vertical loop table creation or by copying cells, merging cells of a table, inserting rows and columns, defining a width for rows, and column and so on.
Add Footnote to a Word Documents
The free docxtemplater library includes support for adding footnotes to a DOCX Word document. The library gives full control for the customization of the footnotes. You can add numbers in superscript and apply different styles to the content of the footnote with ease.
Add Footnote to Documents via JavaScript
const imageOpts = {
getProps: function (img, tagValue, tagName) {
/*
* If you don't want to change the props
* for a given tagValue, you should write :
*
* return null;
*/
return {
rotation: 90,
// flipVertical: true,
// flipHorizontal: true,
};
},
getImage: function (tagValue, tagName) {
return fs.readFileSync(tagValue);
},
getSize: function (img, tagValue, tagName) {
return [150, 150];
},
};
const doc = new Docxtemplater(zip, {
modules: [new ImageModule(imageOpts)],
});
Add and Modify Images in DOCX
The open source docxtemplater library gives software programmers the power to insert images inside a word document. The library allows setting the image width and height, aligning images, adding a caption for the images, using angular expressions to set image sizes, and so on. You can also retrieve image data from any data source such as base64 data, filesystem, URL, and Amazon S3 stored image. One great feature of the library is that you can avoid pictures bigger than their container.
Rotate and Flip Images via JavaScript
const imageOpts = {
getProps: function (img, tagValue, tagName) {
/*
* If you don't want to change the props
* for a given tagValue, you should write :
*
* return null;
*/
return {
rotation: 90,
// flipVertical: true,
// flipHorizontal: true,
};
},
getImage: function (tagValue, tagName) {
return fs.readFileSync(tagValue);
},
getSize: function (img, tagValue, tagName) {
return [150, 150];
},
};
const doc = new Docxtemplater(zip, {
modules: [new ImageModule(imageOpts)],
});