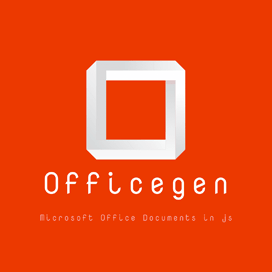
Officegen-DOCX
Open Source JavaScript Library for Word Processing
Create, Modify & Convert Microsoft® Word DOCX Documents via Open Source APIs.
What is Officegen-DOCX?
Officegen-DOCX is an Open Source JavaScript library that allows software developers to work with Office Open XML files and create Word (Docx) for MS Office 2007 & later inside their own JavaScript applications. Officegen-DOCX is very flexible and can be used on several environments. The environments that support Node.js are fully compatible with it, such as Linux, OSX, and Windows.
It also supports several Word processing features for Microsoft Word documents (DOCX file) such as creating Word documents, adding one or more paragraphs, adding images, header and footer support, bookmarks, and hyperlinks support.
Getting Started with Officegen-DOCX
The most recent release of officegen-DOCX can be installed directly from the online Officegen-DOCX repository by applying the following command.
Install using officegen repository
$ npm install Ziv-Barber/officegen#master
Create Word Document via Free JavaScript API
Officegen-DOCX enables software programmers to create a new Word DOCX Document inside JavaScript applications. It also enables developers to alter existing Microsoft Word Documents according to their needs. You also can insert paragraphs, add images, align text or objects, add headers and footer, bookmarks and hyperlinks support, change font styles, and more. The following simple lines of code can create a Word document in JavaScript.
- Instantiate officegen
- Create an empty Word document
- Set output path & save document
Create an Empty DOCX - JavaScript
const officegen = require('officegen')
const fs = require('fs')
// Create an empty Word document
let docx = officegen('docx')
// Set output path
let out = fs.createWriteStream('empty.docx')
// Save
docx.generate(out)
Inserting Images to Word DOCX Files
Officegen-DOCX gives computer programmers the power to add images inside their Word DOCX document in JavaScript applications with few lines of code. For adding an image inside Word documents you need to provide the name as well as the location of the image.
Add Image in DOCX - JavaScript
const officegen = require('officegen')
const fs = require('fs')
// Create a new word document
let docx = officegen('docx')
// Create a new paragraph
let pObj = docx.createP()
// Add Image
pObj.addImage('sample.jpg')
// Set output path
let out = fs.createWriteStream('image.docx')
// Save
docx.generate(out)
Add Paragraphs to Word DOCX Files
Officegen-DOCX enables computer programmers to add content in their Word DOCX files inside their own JavaScript applications. The API support adding one or more paragraphs to word documents. It also facilitates you to set the fonts, colors, alignment etc. for your content with ease.
Add Paragraph in DOCX - JavaScript
const officegen = require('officegen')
const fs = require('fs')
// Create a new word document
let docx = officegen('docx')
// Add Paragraph
let pObj = docx.createP()
// Add Text in it
pObj.addText('FileFormat Developer Guide')
// Set output path
let out = fs.createWriteStream('fileformat.docx')
// Save
docx.generate(out)