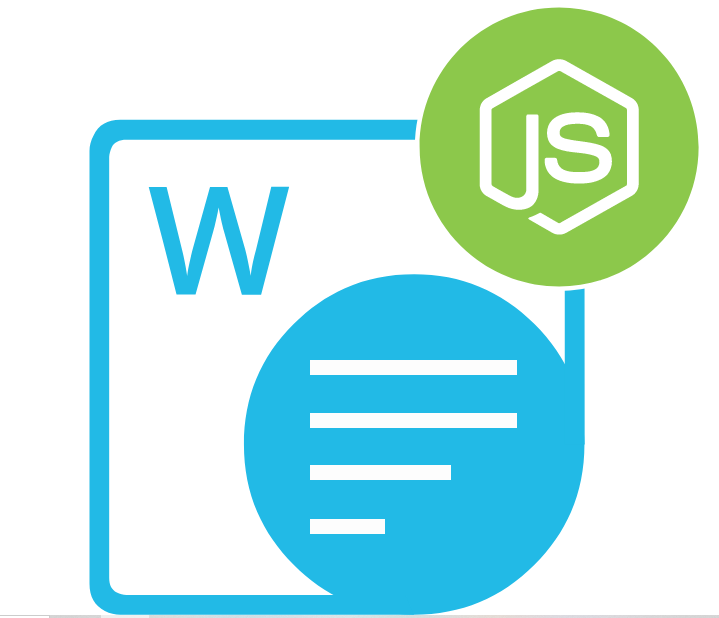
Aspose.Words Cloud SDK for Node.js
Node.js API to Create/Convert Word DOC, DOCX Files
A Powerufl Node.js REST SDK to Generate, Edit, Print, Manipulate, Read, & Convert Word DOC, DOCX Documents without MicroSoft Word, or Office Automation inside Node.js Envrionment.
What is Aspose.Words Cloud SDK for Node.js?
Efficient document processing always plays a vital role in various industries, from legal to healthcare, education, and beyond. As businesses strive for efficiency and accuracy, the need for reliable tools to manipulate and manage documents becomes increasingly apparent. Aspose.Words Cloud SDK for Node.js emerges as a leading and powerful solution, offering a seamless experience for developers to integrate document processing capabilities into their applications.
Aspose.Words Cloud SDK for Node.js is a very important part of the Aspose.Words product family, renowned for its robustness and flexibility in dealing with document formats such as DOCX, DOC, RTF, DOT, DOTX, DOTM, ODT, OTT, WordML, HTML, and more. With its comprehensive set of features, software developers can effortlessly perform a wide range of document-related tasks, from creating, editing, and converting documents to extracting text, splitting or merging pages, manipulating formatting, and much more. The API simplifies the process of generating personalized documents using mail merge functionality.
The Aspose.Words Cloud SDK for Node.js empowers software developers to integrate document manipulation features directly into their Node.js applications, without the need for complex setups or infrastructure management. One of the standout features of the REST API is its support and flexibility in dealing as well as converting Word document to popular formats like RTF, PDF, HTML, ODT, OTT, TXT, EPUB, XPS, PCL, TIFF, PNG, JPEG, BMP, SVG, DOCX, DOCM, DOTX, and many more. With its rich feature set, intuitive API, and robust security measures, the cloud SDK empowers software developers to build innovative applications that manipulate documents with ease and efficiency.
Getting Started with Aspose.Words Cloud SDK for Node.js
To install Aspose.Words Cloud SDK for Node.js, you can use npm, the package manager for JavaScript. Please use the following commands for a successful installation.
Install Aspose.Words for .NET via npm
npm install asposewordscloud
You can download the directly from Aspose.Words Release pageCreate Word Document inside Node.js Apps
Aspose.Words Cloud SDK for Node.js has provided complete functionality that allows software developers to programmatically create a new word document and add contents to it inside their own Node.js applications. After the creation of document developers can easily add text, images, shapes, manage fonts, define styles and formatting, manage page size, insert tables and charts, add headers/footers and so on. Here is a simple example that shows how software developers can create a new word document inside Node.js applications.
How to Create a New Word Documents inside Node.js Apps?
import * as fs from "fs";
const clientId = "####-####-####-####-####";
const secret = "##################";
const wordsApi = new WordsApi(clientId, secret);
const createRequest = new model.CreateDocumentRequest({
fileName: "Sample.docx"
});
wordsApi.createDocument(createRequest)
.then((createRequestResult) => {
// tslint:disable-next-line:no-console
console.log("Result of createRequest: ", createRequestResult);
});
Word Document Conversion via Node.js SDK
Software developers can use Aspose.Words Cloud SDK for Node.js, to convert MS Word documents from one format to another with just a couple of lines of code inside their own applications. Whether you need to convert DOCX to PDF, HTML to DOC, or any other combination, the SDK provides simple methods to accomplish these tasks efficiently. The following example demonstrates how software developers can convert word DOCX file to PDF inside Node.js environment.
How to Convert DOCX to PDF via Node.js?
// create API instance (baseUrl is optional)
const wordsApi = new WordsApi(clientId, clientSecret, baseUrl);
// upload file to the Aspose cloud
const uploadRequest = new UploadFileRequest();
uploadRequest.path = "uploaded.docx";
uploadRequest.fileContent = createReadStream(localPath);
wordsApi.uploadFile(uploadRequest)
.then((_uploadResult) => {
// save the file as pdf in the cloud
const request = new SaveAsRequest({
name: "uploaded.docx",
saveOptionsData: new PdfSaveOptionsData(
{
fileName: "destination.pdf"
})
});
wordsApi.saveAs(request)
.then((_result) => {
// deal with the pdf file
})
.catch(function(_err) {
// handle saveAs request error
});
})
.catch(function(_err) {
// handle uploadFile request error
});
Extract Text from Word Documents via Node.js
Extracting text as well as images from various types of documents is a common requirement in many applications, such as text analysis or content indexing and so on. Aspose.Words Cloud SDK for Node.js offers seamless text extraction capabilities, allowing software developers to retrieve text content with ease. The SDK also provides useful methods for extracting logos, illustrations, or photographs embedded within the document. Here's an example that shows how users can extract text from a DOCX document inside Node.js.
How to Extract Text from a DOCX Document via Node.js?
const { WordsApi, GetDocumentTextRequest } = require("asposewordscloud");
// Initialize Words API
const wordsApi = new WordsApi();
// Extract text from DOCX
const getTextRequest = new GetDocumentTextRequest({
name: "input.docx",
});
wordsApi.getDocumentText(getTextRequest)
.then((result) => {
console.log("Text extracted:", result.text);
})
.catch((error) => {
console.error("Error extracting text:", error);
});
Apply Mail Merge in Node.js
What sets Aspose.Words Cloud SDK apart is its intuitive API design, which allows software developers to perform complex tasks with just a few lines of code. The SDK simplifies the process of generating personalized documents using mail merge functionality inside Node.js applications. Software developers can easily populate document templates with data from external sources, such as databases or JSON files, to create customized documents in bulk. Here's an example of performing a mail merge with a JSON data source.
How to Performing a Mail Merge with a JSON Data Source inside Node.js?
const { WordsApi, ExecuteMailMergeOnlineRequest, MailMergeData } = require("asposewordscloud");
// Initialize Words API
const wordsApi = new WordsApi();
// Perform mail merge with JSON data
const mailMergeData = new MailMergeData();
mailMergeData.dataSourceType = "json";
mailMergeData.dataSource = fs.createReadStream("data.json");
const executeMailMergeRequest = new ExecuteMailMergeOnlineRequest({
template: fs.createReadStream("template.docx"),
data: mailMergeData,
});
wordsApi.executeMailMergeOnline(executeMailMergeRequest)
.then((result) => {
console.log("Mail merge completed. Merged document saved.");
})
.catch((error) => {
console.error("Error performing mail merge:", error);
});