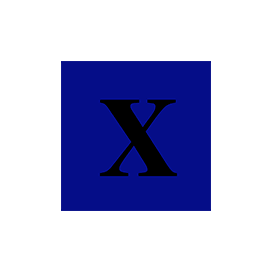
Docxtemplater
Free Node.js Library to Create & Manage Word DOCX from Templates
A Leading Open Source Node.js Library allows to Create, Edit and Manipulate Word DOCX, XLSX Documents Dynamically using a Simple Template-based Approach via JavaScript API .
What is Docxtemplater?
In today's fast-paced digital world, efficiency is paramount, especially when it comes to document management. Whether user’s need to draft contracts, generate reports, or craft personalized letters, having a reliable tool to automate document creation can save them valuable time and resources. Enter Docxtemplater, a powerful library that simplifies the process of generating Word documents from templates. The library enables template-based document generation, where placeholders within a Word document template are replaced with actual data to create the final output document.
At its core, Docxtemplater is a powerful open source JavaScript library that allows software developers to create and manage dynamic Word, Excel and PowerPoint documents by merging data with a pre-defined template. Leveraging the OpenXML format, the library has provided fine-grained control over the structure and content of user’s documents, enabling them to insert placeholders that will be replaced with actual data during runtime.
Docxtemplater is a versatile library that empowers software developers to automate document creation with ease. By leveraging the power of templates and data merging, you can streamline your document workflow and boost productivity significantly. By using the library properly, software developers can easily generate dynamic documents tailored to their specific needs. Whether you're automating document generation tasks or personalizing reports, Docxtemplater is a valuable tool in your arsenal.
How to Install Docxtemplater?
To install Docxtemplater, you can use npm, the package manager for JavaScript. Please use the following commands for a successful installation.
Install Docxtemplater via npm
npm install --save docxtemplater pizzip
Word Documents Creation via Template in Node.js
The open source Docxtemplater library makes it easy for software developers to Generate Microsoft Word DOCX documents using a template. Software developers can inject dynamic data into the template by passing an object containing key-value pairs to replace placeholders with actual data to create the final output document. The following example demonstrates how developers can load an existing template and generate Word document from it inside Node.js environment.
How to Generate Word Document from a Template inside Node.js?
const fs = require('fs');
const Docxtemplater = require('docxtemplater');
// Load the template
const content = fs.readFileSync('template.docx', 'binary');
const doc = new Docxtemplater(content);
// Set data to fill placeholders
const data = {
firstName: 'John',
lastName: 'Doe'
};
// Replace placeholders with actual data
doc.setData(data);
// Render the document
doc.render();
// Save the generated document
const output = fs.writeFileSync('output.docx', doc.getZip().generate({type: 'nodebuffer'}));
console.log('Document generated successfully!');
Add Custom Functions & Filters in Node.js Apps
Custom Functions and Filters in Docxtemplater library offer advanced capabilities for manipulating data and performing specific operations within templates. They enable users to extend the functionality of the library beyond basic placeholder replacement, allowing for dynamic content generation and complex data processing. You can define custom functions and filters to manipulate data or perform specific operations within your templates. Here is an example that shows developers can implement a custom function to calculate the total price of items in a shopping cart and a filter to format currency values.
How to Calculate Prices Items in a Shopping Cart via Custom Function & Use Filters to Format Currency Value?
const fs = require('fs');
const Docxtemplater = require('docxtemplater');
// Define custom function to calculate total price
function calculateTotal(items) {
return items.reduce((total, item) => total + item.price * item.quantity, 0);
}
// Define custom filter to format currency
function formatCurrency(value) {
return '$' + value.toFixed(2); // Format as dollars with 2 decimal places
}
// Load the template
const content = fs.readFileSync('template.docx', 'binary');
const doc = new Docxtemplater(content, {
parser: {
// Define custom tag for invoking functions
getFunction: function(tag) {
if (tag === 'calculateTotal') {
return calculateTotal;
}
},
// Define custom tag for applying filters
getFilter: function(tag) {
if (tag === 'currency') {
return formatCurrency;
}
}
}
});
// Set data with shopping cart items
const data = {
items: [
{ name: 'Product 1', price: 10, quantity: 2 },
{ name: 'Product 2', price: 20, quantity: 1 },
{ name: 'Product 3', price: 15, quantity: 3 }
]
};
// Replace placeholders with actual data
doc.setData(data);
// Render the document
doc.render();
// Save the generated document
const output = fs.writeFileSync('output.docx', doc.getZip().generate({ type: 'nodebuffer' }));
console.log('Document generated successfully!');
Rich Formatting Support
The open source Docxtemplater simplifies the process of creating and managing Word documents by offering a straightforward template-based approach. Rich formatting support in Word documents refers to the ability to apply various styling attributes such as font styles, colors, sizes, alignments, and more to different elements within the Word document. This includes formatting text, tables, images, paragraph, section formatting, list, bullet points and other content to enhance readability and visual appeal. Here is a simple example that shows how to apply Rich Formatting to a part of text inside a document.
How to apply rich formatting to text using Docxtemplater in a Node.js application?
// Render the document with custom parser options for rich formatting
doc.render({
parser: {
// Custom tag for interpreting HTML-like tags for rich formatting
tagToken: function(tag) {
return {
tagName: tag.substring(1),
mode: 'open'
};
},
// Apply rich formatting based on tag names
commands: {
b: function(scope, context, tag) {
return {
type: 'applyRichText',
value: { b: true }
};
},
i: function(scope, context, tag) {
return {
type: 'applyRichText',
value: { i: true }
};
},
u: function(scope, context, tag) {
return {
type: 'applyRichText',
value: { u: true }
};
},
strike: function(scope, context, tag) {
return {
type: 'applyRichText',
value: { strike: true }
};
}
}
}
});
// Save the generated document
const output = fs.writeFileSync('output.docx', doc.getZip().generate({ type: 'nodebuffer' }));
console.log('Document generated successfully!');