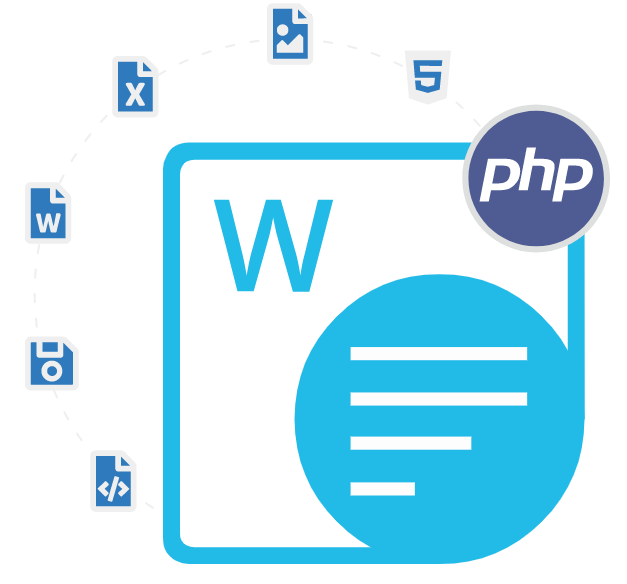
Aspose.Words Cloud SDK for PHP
PHP API to Create & Process Microsoft Word Documents
REST API for Creating, Editing, Splitting, Merging, Manipulating, and Converting Word (DOC, DOCX, ODT) to PDF, HTML, EPUB, Images & more in PHP Apps.
Aspose.Words Cloud SDK for PHP is like a tool in the cloud that helps developers add cool features for working with Word documents to their PHP apps. It’s a smart way to handle Word docs without spending too much time or money. This SDK lets you make, tweak, and change Word files easily. You can also manage stuff like who wrote the doc, its title, and keywords. The PHP Cloud SDK acts as a bridge to the Cloud API. It’s made to be easy, fast, safe, and boost your productivity. Software Developers can easily incorporate Word document generation, editing, and conversion facilities into PHP applications running on any modern OS.
The Aspose.Words Cloud SDK for PHP is a user-friendly and robust tool that offers various essential features for creating and handling word documents. It allows you to do things like add and modify bookmarks, search for specific text within documents, replace text with different content, include comments, retrieve and update comments, add new pages, compress documents, handle DrawingObjects, insert fields, and manage headers and footers, insert & update footnotes, insert and manage tables, perform Mail Merge online, get all fonts in a Word document, among other functions.
With the Aspose.Words Cloud SDK for PHP, you can easily convert documents to various popular file types or switch between different formats effortlessly. This SDK lets you convert to over 20 file formats like EMF, PDF, HTML, EPUB, DOTX, GIF, BMP, PNG, RTF, XPS, WML, and more. You can also utilize the SDK to carry out mail merge tasks, like crafting letters, envelopes, and labels from databases or other data sources.
Getting Started with Aspose.Words Cloud PHP REST API
Download, install and configure PHP 7.1 or higher version on your web-server. The recommend way to install Aspose.Words Cloud PHP REST API is using Composer. Please use the following command for a smooth installation.
Install Aspose.Words Cloud PHP REST API via Composer
composer require aspose-cloud/aspose-words-cloud
You can also download it directly from GitHub.Generate & Manage Word Documents via PHP REST API
Aspose.Words Cloud PHP REST API has provided complete support for creating new Word documents from the scratch inside PHP applications. The library fully supports several basic as well as advanced features for Word documents creation and processing such as add new pages, compress a Word document online, access and modify document properties, get statistics on a Word document, protect word documents, render parts of a Word document into an image, remove all macros from a Word document and many more.
Create New Word Documents via PHP API
<php
use Aspose\Words\WordsApi;
use Aspose\Words\Model\Requests\{CreateDocumentRequest};
$clientId = '####-####-####-####-####';
$secret = '##################';
$wordsApi = new WordsApi($clientId, $secret);
$createRequest = new CreateDocumentRequest(
"Sample.docx", NULL, NULL);
$wordsApi->createDocument($createRequest); ?>
Convert Word Documents using PHP REST API
Aspose.Words Cloud PHP REST API allows software developers to convert Microsoft Word and OpenOffice documents to other supported file formats with high quality and speed. The library supports conversion to various popular file formats such as DOCX, DOC, RTF, ODT, PDF, HTML, MD, XAML, TXT, PNG, JPG and many others. The library also included various other popular documents conversions such as, Convert PDF to Word, Convert Word to PDF, Convert MOBI to EPUB, Convert MOBI to PDF, Convert Word to EPUB, Convert Word to Image, Convert Word to HTML and many more.
How to convert PDF to Word Programmatically via PHP REST API?
<php
use Aspose\Words\WordsApi;
use Aspose\Words\Model\Requests\{SaveAsOnlineRequest};
use Aspose\Words\Model\{SaveOptionsData};
$clientId = '####-####-####-####-####';
$secret = '##################';
$wordsApi = new WordsApi($clientId, $secret);
$requestDocument = "Sample.docx";
$requestSaveOptionsData = new SaveOptionsData(array(
"save_format" => "pdf",
"file_name" => "Sample_out.pdf",));
$saveRequest = new SaveAsOnlineRequest(
$requestDocument, $requestSaveOptionsData, NULL, NULL, NULL, NULL);
$wordsApi->saveAsOnline($saveRequest); ?>
Comparing Multiple Word Documents via PHP
Aspose.Words Cloud SDK for PHP enables software developers to compare their Word documents with just couple of lines of PHP code. The library allows programmatically comparing two Word documents and retrieving the differences between them. The library supports displaying the result of the documents comparison and can include information about the type of difference such as updated text, deleted text, newly added text, formatting change and so on. It may provide information about the location of the difference in the document, and the text that was added or deleted.
Generate Report via PHP library
Aspose.Words Cloud SDK for PHP has provided complete functionality for generating professional print-ready DOCX, DOC, RTF, PDF reports from templates inside PHP applications. To generate a report, first you need to prepare your source data in JSON, XML or CSV formats, then Create a report template and write a simple PHP application to bind the data to the template and produce the output document.
Run Report Generation Process using PHP Commands
<php
use Aspose\Words\WordsApi;
use Aspose\Words\Model\Requests\{BuildReportOnlineRequest};
use Aspose\Words\Model\{ReportEngineSettings};
$clientId = '####-####-####-####-####';
$secret = '##################';
$wordsApi = new WordsApi($clientId, $secret);
$requestTemplate = "Sample.docx";
$requestReportEngineSettings = new ReportEngineSettings(array(
"data_source_type" => "Json",
"data_source_name" => "persons",));
$buildReportRequest = new BuildReportOnlineRequest(
$requestTemplate, "Data.json", $requestReportEngineSettings, NULL);
$wordsApi->buildReportOnline($buildReportRequest); ?>