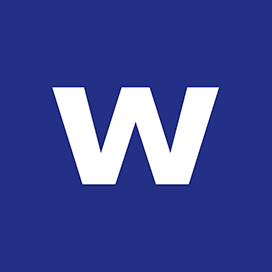
PHPWord
Open Source PHP Library for Microsoft® Word Documents
Read, Write, Protect, Process and Convert MS Word DOC, DOCX, ODT, RTF & HTML files to PDF file format via Open Source Free PHP APIs.
What is PHPWord?
PHPWord is an open-source library that consists of classes to assist you in developing PHP applications that can interact with various document file formats. PHPWord is licensed under LGPL Version 3 and enables you to work with document settings, styles, templates, and various other elements.
PHPWord offers a range of features that make it an essential library for developers looking to work with Word documents programmatically, such as create complex Word documents with a wide range of formatting options, protecting word documents from unauthorized access, creating and managing tables in Word files, insertion of images and other media types into documents, and advanced content elements support such as lists (both ordered and unordered), hyperlinks, footnotes, endnotes and many more.
Getting Started with PHPWord
To create a word document using the PHPWord you need the following resources installed in your operating system:
- PHP version 5.3.3+
- Composer
- XML Parser Extension( This extension is enabled by default )
- Zend Escaper Component Install it using composer require zendframework/zend-escaper
Create Word Document using PHPWord
PHPWord allows the developers to create new Word Document (DOCX) from scratch. It allows you to add new paragraphs, titles, text, images, hyperlinks, charts and more. Creating a word document is simple, you need to create a new document using PhpWord() method.
Create Word in PHP
- Create word document using PhpWord
- Add section in document
- Add text in section
- Save document
Create a Word Document - PHP
<?php
require_once 'vendor\phpoffice\phpword\bootstrap.php';
// Create the new document..
$phpWord = new \PhpOffice\PhpWord\PhpWord();
// Add an empty Section to the document
$section = $phpWord->addSection();
// Add Text element to the Section
$section->addText(
'File Format Developer Guide - '
. 'Learn about computer files that you come across in '
. 'your daily work at: www.fileformat.com'
);
// Save document
$objWriter = \PhpOffice\PhpWord\IOFactory::createWriter($phpWord, 'Word2007');
$objWriter->save('HelloWorld.docx');
Configure Document Settings with Ease
You can perform various settings of documents. The default magnification for the document is 100 percent but you can change it to some other value. For double-sided documents, e.g. magazines, you can set up facing pages by mirroring the page margins. You can tweak the status of grammatical or spelling errors auto-check option.
Feature to track document revisions is also available. PHPWord can be configured to work with Latin languages, East Asian languages as well as Complex (Bi-Directional) languages. You can protect a document or its part with a password.
There are a lot of other features that PHPWord allows you to configure for documents, some of those include, document information, measure units, hyphenation, and automatic recalculation of document fields whenever the document is opened.
Set DOCX Properties - PHP
<?php
require_once 'vendor\phpoffice\phpword\bootstrap.php';
// Create the new document..
$phpWord = new \PhpOffice\PhpWord\PhpWord();
// Set document properties
$properties = $phpWord->getDocInfo();
$properties->setCreator('Ali Ahmed');
$properties->setCompany('File Format');
$properties->setTitle('PHPWord');
$properties->setDescription('File Format Developer Guide');
$properties->setCategory('My category');
$properties->setLastModifiedBy('My name');
$properties->setCreated(mktime(0, 0, 0, 3, 12, 2019));
$properties->setModified(mktime(0, 0, 0, 3, 14, 2019));
$properties->setSubject('PHPWord');
// Save document
$objWriter = \PhpOffice\PhpWord\IOFactory::createWriter($phpWord, 'Word2007');
$objWriter->save('DocumentProperties.docx');
Containers to Organize Document Elements
PHPWord has objects called containers, which hold various elements (tables, text, etc) of a document. There are 3 primary containers; sections, headers, and footers. Also, there are 3 elements that can act as containers; textures, table cells, and footnotes.
All the visible elements of a document need to be placed inside a section. You can assign page numbers, line numbers, change the layout to multi-column, and create headers/footers.
Add Header Container
<?php
require_once 'vendor\phpoffice\phpword\bootstrap.php';
// Create the new document..
$phpWord = new \PhpOffice\PhpWord\PhpWord();
// Add an empty Section to the document
$section = $phpWord->addSection();
// Add Header
$header = $section->addHeader();
$header->addImage('word-processing-image.png');
// Save document
$objWriter = \PhpOffice\PhpWord\IOFactory::createWriter($phpWord, 'Word2007');
$objWriter->save('Container.docx');
Protect Word Document via PHPWord API
It is always wise to protect or secure your important documents which encompass sensitive information. The open-source PHPWord library enables Software developers to protect their Word documents by providing a unique password inside their PHP applications. The protection will work as a safeguard, preventing unauthorized access or changes to the Word document.