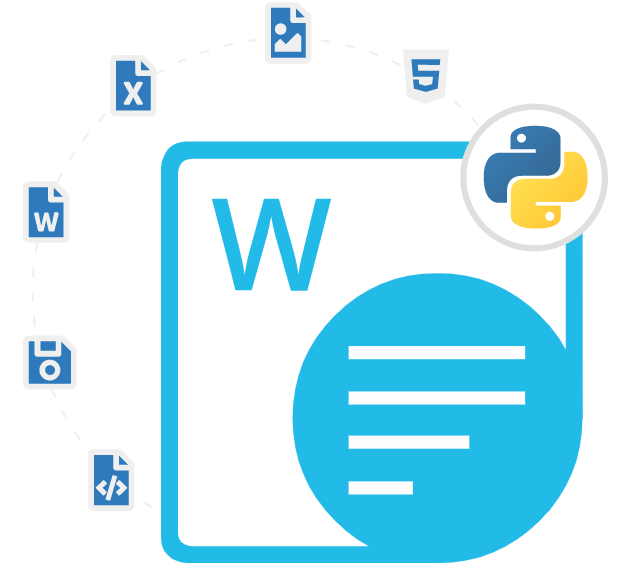
Aspose.Words Cloud SDK for Python
Python REST SDK to Process Microsoft Word Documents
Python Cloud API allows to create, manipulate, and convert Microsoft Word documents to PDF to Word (DOC, DOCX, ODT, RTF and HTML).
Aspose.Words Cloud SDK for Python is a very useful software development kit (SDK) that gives developers the simplest and efficient ways for working with Microsoft Word documents in the cloud. The Python Cloud SDK is a high-level wrapper around the Aspose.Words API, providing Python developers with a powerful and intuitive way to use the API inside their cloud-based applications. It provides a set of Python classes and methods that enable developers to create, modify, and convert Word documents in the cloud.
The Python Cloud SDK has included a several important features and capabilities that enable software developers to perform a wide range of operations on Word documents, including reading and writing Word documents in various formats, manipulating the contents of Word documents, generating various types of repots & templates, converting Word documents to other formats, adding watermarks, inserting headers and footers to Word documents, Encrypting and decrypting Word files, applying mail merge operation, merging multiple files into one, splitting large files, and many more.
Aspose.Words Cloud SDK for Python has included a very powerful document conversion features that allows developers to convert Word documents to different file formats including DOC, DOT, DOCX, DOCM, DOTX, DOTM, RTF, PDF, HTML, ODT, OTT, TXT, EPUB, XPS, PCL, TIFF, PNG, JPEG, BMP, SVG and may more. The SDK also provides a range of other options and settings that enable developers to customize the creation and formatting of Word documents in the cloud.
Getting Started with Aspose.Words Cloud SDK for Python
Firstly, create an account at Aspose for Cloud to get your application information and free quota to use the API. Now execute pip install aspose-words-cloud from the command line to fetch the SDK. Then import the package via import asposewordscloud.
Install Aspose.Words Cloud SDK for Python via Setuptools
python setup.py install --user
// import the package
import asposewordscloud
You can also download it directly from GitHub.Create Word Document via Python API
Aspose.Words Cloud for Python gives software developers the capability to create new Word processing documents from the scratch inside their own Python applications. The Library has included several important features related to documents creation and management, such as insert new pages to an existing documents, insert images to word documents, update documents properties, split a large documents to smaller ones, split word documents, merge documents, protect word documents by applying password, compare documents for differences, and many more.
Create Word Documents via Python API
import os
import asposewordscloud
import asposewordscloud.models.requests
from asposewordscloud.rest import ApiException
from shutil import copyfile
words_api = WordsApi(client_id = '####-####-####-####-####', client_secret = '##################')
create_request = asposewordscloud.models.requests.CreateDocumentRequest(file_name='Sample.docx')
words_api.create_document(create_request)
Convert Word Document via Python API
Aspose.Words Cloud for Python gives software developers the power to convert Word documents from one format to another format with just a couple of lines of Python code. The library allows import and export documents in various documents formats such as DOC, DOCX, PDF, RTF, DOT, DOTX, ODT, OTT, HTML, MHTML, XML and TXT. It also provided export-only to some popular file formats such as PS, XPS, OpenXPS, PNG, JPEG, BMP, SVG, TIFF, EMF, PCL, EPUB and many more.
Convert Word Documents to Other Formats via Python API
import os
import asposewordscloud
import asposewordscloud.models.requests
from asposewordscloud.rest import ApiException
from shutil import copyfile
words_api = WordsApi(client_id = '####-####-####-####-####', client_secret = '##################')
request_document = open('Sample.docx', 'rb')
convert_request = asposewordscloud.models.requests.ConvertDocumentRequest(document=request_document, format='pdf')
words_api.convert_document(convert_request)
Manage Headers/Footers & Hyperlinks via Python API
Aspose.Words Cloud SDK gives software developers ability to manage Headers and Footers inside word documents using Python commands. The library has included supports for inserting a Header/Footer into a Word document, update content of headers/footers, get a Header/Footer of a Word document as well as of a section, link headers/footers of a section to the previous one, delete a `HeaderFooter` object from a Word document online and many more. Developers also add, delete and get hyperlinks from word documents with ease.
Insert a Header/Footer into Word Document via Python API
import os
import asposewordscloud
import asposewordscloud.models.requests
from asposewordscloud.rest import ApiException
from shutil import copyfile
words_api = WordsApi(client_id = '####-####-####-####-####', client_secret = '##################')
request_document = open('Sample.doc', 'rb')
insert_request = asposewordscloud.models.requests.InsertHeaderFooterOnlineRequest(document=request_document, section_path='', header_footer_type='FooterEven')
words_api.insert_header_footer_online(insert_request)
Work with Tables inside Word Documents via Python SDK
Aspose.Words Cloud for Python makes it easy for software developers to insert and update tables inside Microsoft Word documents using Python API. The library has included several important features for handling tables in Word documents such as, inserting rows into a table, Insert a cell into a given row of a table online, delete rows or columns from a table, Get a table border, Get all tables in a Word document, Get the formatting properties of a table row, Get table properties in a Word document online, merge rows or columns, Updates the formatting properties of a table cell, update the border properties of a table and many more.
Insert a Row into a Table inside Word Document via Python
import os
import asposewordscloud
import asposewordscloud.models.requests
from asposewordscloud.rest import ApiException
from shutil import copyfile
words_api = WordsApi(client_id = '####-####-####-####-####', client_secret = '##################')
request_document = open('Sample.docx', 'rb')
request_row = asposewordscloud.TableRowInsert(columns_count=5)
insert_request = asposewordscloud.models.requests.InsertTableRowOnlineRequest(document=request_document, table_path='sections/0/tables/2', row=request_row)
words_api.insert_table_row_online(insert_request)