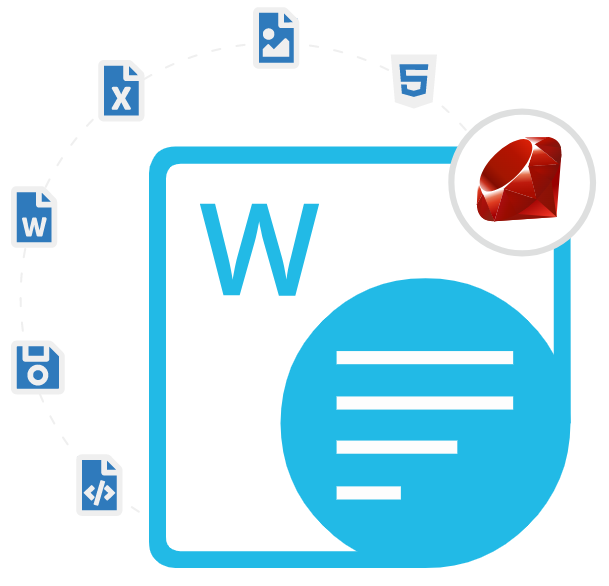
Aspose.Words Cloud Ruby SDK
Ruby SDK to Create & Convert MS Word Documents
Ruby Cloud API to Generate, Edit, Split, Merge, Manipulate, and Convert Word (DOC, DOCX, ODT) to PDF, HTML, EPUB, images & many more.
Aspose.Words Cloud SDK for Ruby is a powerful library that enables software programmers to work with Microsoft Word documents in the cloud. This SDK provides a complete set of features for creating, manipulating, and converting Word documents. The Aspose.Words Cloud SDK for Ruby is built on top of the Aspose.Words Cloud REST API, which is an advanced and reliable API for working with Microsoft Word documents in the cloud.
Aspose.Words Cloud SDK for Ruby has provided a comprehensive set of features for working with Word documents. It allows Software Developers to generate new documents from scratch, modify existing documents, convert Word documents to other supported file formats, manipulate document properties, split large documents into smaller ones, combine multiple documents, add watermarks in Word documents, apply a password to word documents, execute Mail Merge, add and manage tables in documents, compare Word documents, insert image and graphics into Word documents and many more.
Aspose.Words Cloud SDK for Ruby provides an easy-to-use interface for Ruby developers, making it simple to integrate the cloud-based Word processing capabilities into their applications and helping them to encrypt and protect their input documents. It provides supports a wide range of Word document formats, including DOC, DOT, DOCX, DOCM, DOTX, DOTM, RTF, PDF, HTML, ODT, OTT, TXT, EPUB, XPS, PCL, TIFF, PNG, JPEG, BMP, SVG and many more. Overall, the Aspose.Words is a powerful tool for Ruby developers who need to work with Word documents in the cloud.
Getting Started with Aspose.Words Cloud SDK for Ruby
The recommend way to install Aspose.Words Cloud SDK for Rubyis using RubyGem. Please use the following command for a smooth installation.
Install Aspose.Words Cloud SDK for Ruby via RubyGem
//To install this package do the following: update your Gemfile
gem 'aspose_words_cloud', '~> 23.2'
// or install directly
gem install aspose_words_cloud
You can also download it directly from GitHub.Word Documents Generation via Ruby Cloud API
Aspose.Words Cloud SDK for Ruby gives software programmers the capability to generate new Microsoft Word and OpenOffice documents without installing Microsoft Office. It can create Word files in DOC, DOCX, RTF, DOT, DOTX, DOTM, FlatOPC (XML), and more. The library has included various features for handling Word document creation and management, such as Inserting new pages, adding watermarks in Word documents, Inserting a page number field, adding and manging tables, inserting text to Word documents, document & text classifications, and many more.
Create a New Word Document in Python Applications
require 'aspose_words_cloud'
AsposeWordsCloud.configure do |config|
config.client_data['ClientId'] = '####-####-####-####-####'
config.client_data['ClientSecret'] = '##################'
end
@words_api = WordsAPI.new
create_request = CreateDocumentRequest.new(file_name: 'Sample.docx')
@words_api.create_document(create_request)
Convert Word Documents via Ruby API
Aspose.Words Cloud SDK for Ruby has provided a very powerful feature for converting Word documents to various other supported file formats using RESTAPIs. The library has provided support for importing and exporting documents to some popular file formats like DOC, DOCX, PDF, RTF, DOT, DOTX, ODT, OTT, HTML, MHTML, XML, and TXT. Developers can also export-only Word documents to file formats like PS, XPS, OpenXPS, PNG, JPEG, BMP, SVG, TIFF, EMF, PCL, EPUB, and many more. The following example demonstrates how programmatically software developers can convert Word to PDF.
Convert Word to PDF via Ruby API
require 'aspose_words_cloud'
AsposeWordsCloud.configure do |config|
config.client_data['ClientId'] = '####-####-####-####-####'
config.client_data['ClientSecret'] = '##################'
end
@words_api = WordsAPI.new
request_document = File.open('Sample.docx')
convert_request = ConvertDocumentRequest.new(document: request_document, format: 'pdf')
@words_api.convert_document(convert_request)
Manage Headers/Footers & Hyperlinks via Python API
Aspose.Words Cloud SDK gives software developers ability to manage Headers and Footers inside word documents using Python commands. The library has included supports for inserting a Header/Footer into a Word document, update content of headers/footers, get a Header/Footer of a Word document as well as of a section, link headers/footers of a section to the previous one, delete a `HeaderFooter` object from a Word document online and many more. Developers also add, delete and get hyperlinks from word documents with ease.
Insert a Header/Footer into Word Document via Python API
import os
import asposewordscloud
import asposewordscloud.models.requests
from asposewordscloud.rest import ApiException
from shutil import copyfile
words_api = WordsApi(client_id = '####-####-####-####-####', client_secret = '##################')
request_document = open('Sample.doc', 'rb')
insert_request = asposewordscloud.models.requests.InsertHeaderFooterOnlineRequest(document=request_document, section_path='', header_footer_type='FooterEven')
words_api.insert_header_footer_online(insert_request)
Word Documents Protection in Ruby Applications
Aspose.Words Cloud SDK for Ruby makes it easy for software developers to secure and protect Word documents inside their own Ruby applications. The library has included various features for handling Documents protection, such as adding protection to Word documents, restricting documents editing, encrypting word documents with Password, adding a Digital Signature, and many more. The following example demonstrates how to add protection to a Word document in Cloud.
How to Add Protection to Word Documents via Cloud API
require 'aspose_words_cloud'
AsposeWordsCloud.configure do |config|
config.client_data['ClientId'] = '####-####-####-####-####'
config.client_data['ClientSecret'] = '##################'
end
@words_api = WordsAPI.new
request_document = File.open('Sample.docx')
request_protection_request = ProtectionRequest.new({:NewPassword => '123'})
protect_request = ProtectDocumentOnlineRequest.new(document: request_document, protection_request: request_protection_request)
@words_api.protect_document_online(protect_request)
Merge & Split Word Documents via Ruby API
Aspose.Words Cloud SDK for Ruby has included complete support for splitting and merging Word documents inside their own Ruby applications. The library has included support for merging several Word or PDF documents into a single document with just a couple of lines of code. The library can also Split large documents, split specific Word pages to PDFs or any other support file formats, such as DOC, DOT, DOCX, DOTX, RTF, ODT, OTT, TXT documents, and save the results to DOC, DOCX, PDF, ODT, RTF, HTML, JPEG, PNG, and many other file formats.
How to Split DOCX to PDFs via Ruby Library?
require 'aspose_words_cloud'
AsposeWordsCloud.configure do |config|
config.client_data['ClientId'] = '####-####-####-####-####'
config.client_data['ClientSecret'] = '##################'
end
@words_api = WordsAPI.new
request_document = File.open('Sample.docx')
split_request = SplitDocumentOnlineRequest.new(document: request_document, format: 'text', dest_file_name: 'SplitDocument.text', from: 1, to: 2)
@words_api.split_document_online(split_request)