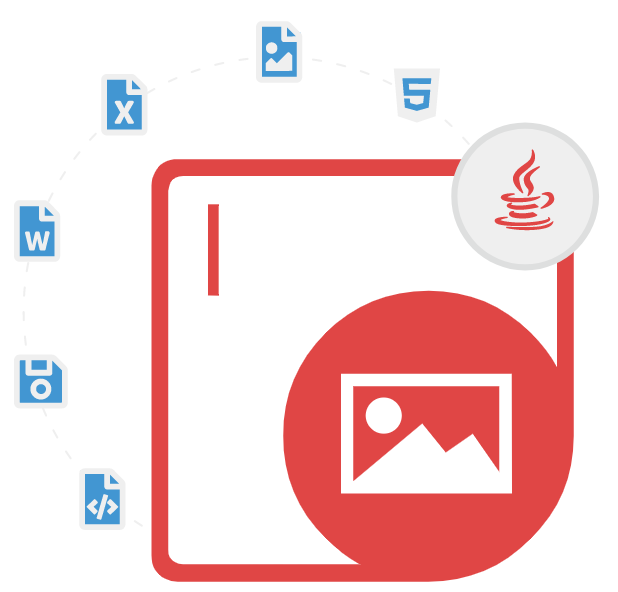
Aspose.Imaging for Java
Java API 来生成、修改和转换图像
实用的 Java API 使软件开发人员能够创建、压缩、编辑、加载、处理图像并将其转换为 JPEG、BMP、TIFF、GIF、PNG 等.
Aspose.Imaging for Java 是一个功能强大的图像处理库,允许软件开发人员在其 Java 应用程序中执行各种图像处理任务。该库允许用户轻松处理图像、在各种图像格式之间转换、轻松执行图像大小调整、裁剪和其他修改。该库已包含对 SVG 和 EMF 等矢量图像的支持。它支持将矢量图像转换为光栅图像、从矢量图像中提取信息等。
Aspose.Imaging for Java 使软件开发人员能够轻松执行复杂的图像处理任务,节省时间和精力。该库支持优化图像以获得更好的质量和更小的文件大小。它提供调整图像亮度、对比度和伽玛以及调整索引图像调色板的功能。该库还支持对 JPEG 和 PNG 等格式的图像进行无损和有损压缩。它提供了读取和写入图像各种属性的功能,例如日期和时间、相机制造商和型号、曝光时间等。
Aspose.Imaging for Java 是一个全面的图像处理库,提供广泛的图像处理、转换、优化和元数据管理功能,例如绘制图像、图像转换为 PDF、矢量图像到矢量化 PSD 图像转换、设置透明图像、将矢量图像转换为矢量化 PSD 图像、保存透明图像、将透明 TIFF 导出为透明 PNG、将 Webp 导出为 PNG、从图像中删除背景、合并图像、将 WMF 和 EMF 转换为其他图像格式、绘制矢量图像等等。
开始使用 Aspose.Imaging for Java
推荐通过 Maven 存储库安装 Aspose.Imaging for Java。您只需在 Maven 项目中通过简单的配置即可轻松使用 Aspose.Imaging for Java API。
Aspose.Imaging for Java 的 Maven 存储库
//First you need to specify Aspose Repository configuration / location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
//Define Aspose.PDF for Java API Dependency
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging</artifactId>
<version>22.12</version>
<classifier>22.12</classifier>
</dependency>
</dependencies>
您可以直接从Aspose.Imaging产品页面下载该库
在 Java 应用程序中生成和编辑图像
Aspose.Imaging for Java 使软件开发人员能够仅用几行 Java 代码从头开始创建新图像。该库提供了几个用于创建和管理图像的重要类。它支持创建各种格式的图像的众多选项,例如 BMP、GIF、JPEG、PNG、TIFF、PSD、DICOM、TGA、ICO、EMZ、WMZ 等等。此外,您可以通过设置路径、通过流创建图像、调整图像大小、在图像上绘制对象、更新图像内容和将图像保存到磁盘、调整图像亮度、对图像应用对比度或伽玛、对图像应用模糊效果、检查图像透明度等来创建图像。
通过 Java API 设置路径来创建图像?
// The path to the documents directory.
String dataDir = "D:/dataDir/";
// Creates an instance of BmpOptions and set its various properties
BmpOptions imageOptions = new BmpOptions();
imageOptions.setBitsPerPixel(24);
// Define the source property for the instance of BmpOptions Second boolean parameter determines if the file is temporal or not
imageOptions.setSource(new FileCreateSource(dataDir + "CreatingAnImageBySettingPath_out.bmp", false));
try
{
// Creates an instance of Image and call Create method by passing the BmpOptions object
try (Image image = Image.create(imageOptions, 500, 500))
{
image.save(dataDir + "CreatingAnImageBySettingPath1_out.bmp");
}
}
finally
{
imageOptions.close();
}
通过 Java API 将图像转换为其他格式
Aspose.Imaging for Java 使软件开发人员能够使用 Java 命令将不同类型的图像转换为其他受支持的文件格式。该库提供了多种将图像从一种格式转换为另一种格式的功能,包括 JPEG、BMP、TIFF、GIF、PNG、DICOM、TGA、ICO、EMZ、WMZ、WebP、SVG 等。该库还支持将图像转换为多页 TIFF、将 TIFF 的各个页面保存为单独的图像以及将图像转换为 PDF。
通过 Java API 将 TIFF 转换为 JPEG 图像
// The path to the documents directory.
String dataDir = Utils.getSharedDataDir(ConvertTIFFToJPEG.class) + "ManipulatingJPEGImages/";
TiffImage tiffImage = (TiffImage)Image.load(dataDir + "source2.tif");
try
{
int i = 0;
for (TiffFrame tiffFrame : tiffImage.getFrames())
{
JpegOptions saveOptions = new JpegOptions();
saveOptions.setResolutionSettings(new ResolutionSetting(tiffFrame.getHorizontalResolution(), tiffFrame.getVerticalResolution()));
TiffOptions frameOptions = tiffFrame.getFrameOptions();
if (frameOptions != null)
{
// Set the resolution unit explicitly.
switch (frameOptions.getResolutionUnit())
{
case TiffResolutionUnits.None:
saveOptions.setResolutionUnit(ResolutionUnit.None);
break;
case TiffResolutionUnits.Inch:
saveOptions.setResolutionUnit(ResolutionUnit.Inch);
break;
case TiffResolutionUnits.Centimeter:
saveOptions.setResolutionUnit(ResolutionUnit.Cm);
break;
default:
throw new RuntimeException("Current resolution unit is unsupported!");
}
}
String fileName = "source2.tif.frame." + (i++) + "."
+ ResolutionUnit.toString(ResolutionUnit.class, saveOptions.getResolutionUnit()) + ".jpg";
tiffFrame.save(dataDir + fileName, saveOptions);
}
}
finally
{
tiffImage.close();
}
通过 Java API 处理图像
Aspose.Imaging for Java 使计算机程序员能够轻松访问和处理现有图像。库中有多种函数用于处理图像,例如更新图像属性、绘制矢量图形、多页图像处理、删除或更新图像背景、图像合并(JPG 到 JPG、JPG 到 PDF 合并、JPG 到 PNG)、图像裁剪、旋转图像、调整图像大小、校正图像倾斜、为图像添加水印、在矢量图像上绘制光栅图像等。
通过 Java API 对图像应用中值滤波器
// Load the noisy image
Image image = Image.load(dataDir + "aspose-logo.gif");
// caste the image into RasterImage
RasterImage rasterImage = (RasterImage) image;
if (rasterImage == null)
{
return;
}
// Create an instance of MedianFilterOptions class and set the size.
MedianFilterOptions options = new MedianFilterOptions(4);
// Apply MedianFilterOptions filter to RasterImage object.
rasterImage.filter(image.getBounds(), options);
// Save the resultant image
image.save(dataDir + "median_test_denoise_out.gif");
通过 Java API 旋转和调整图像大小
Aspose.Imaging for Java 允许软件开发人员以编程方式在自己的 Java 应用程序中旋转和调整图像大小。裁剪是一种非常有用的技术,可用于剪切图像的某些部分以增加对特定区域的关注。该库提供了与图像旋转和调整大小相关的几个功能,例如通过移位裁剪图像、通过矩形裁剪图像、矢量图像裁剪、以 90/180/270 度旋转图像、水平或垂直翻转图像、以指定角度旋转图像、调整 webp 图像大小、按比例调整图像大小等等。
如何通过 Java API 以 Shifts 方式裁剪图像?
u// The path to the documents directory.
String dataDir = "dataDir/jpeg/";
// Load an existing image into an instance of RasterImage class
try (RasterImage rasterImage = (RasterImage)Image.load(dataDir + "aspose-logo.jpg"))
{
// Before cropping, the image should be cached for better performance
if (!rasterImage.isCached())
{
rasterImage.cacheData();
}
// Define shift values for all four sides
int leftShift = 10;
int rightShift = 10;
int topShift = 10;
int bottomShift = 10;
// Based on the shift values, apply the cropping on image Crop method will shift the image bounds toward the center of image and Save the results to disk
rasterImage.crop(leftShift, rightShift, topShift, bottomShift);
rasterImage.save(dataDir + "CroppingByShifts_out.jpg");
}