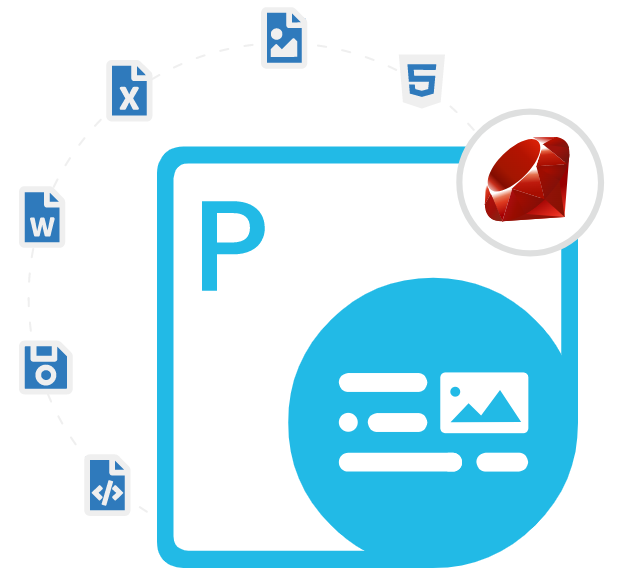
Aspose.PDF Cloud Ruby SDK
用于 PDF 创建和转换的 Python REST API
通过 Python 应用程序内的 Python REST API 生成、编辑、保护、处理、拆分、合并、操作 PDF 文档并将其转换为其他格式,而无需使用 Adobe Acrobat。
Aspose.PDF Cloud Ruby SDK 是一款高级工具,使软件开发人员能够在 云端。 Ruby 软件开发工具包使程序员可以轻松创建强大的应用程序,使用简单直接的 API 调用来创建、编辑、操作和转换 PDF 文件。 该 SDK 与平台无关,可以在任何支持 Ruby 的操作系统或编程语言上使用。 它可用于创建不同格式的 PDF 文档,例如空 PDF、HTML、XML、PCL、SVG、XPS、JPEG、TIFF 等。
Aspose.PDF Cloud Ruby SDK 非常易于处理,并提供了处理 PDF 文件的广泛功能,例如从头开始创建 PDF 文件、从现有(Microsoft Word、Excel 或 PowerPoint)文档生成 PDF, PDF 编辑选项、添加或删除新的 PDF 页面、在 PDF 中插入或删除文本、在 PDF 文件中插入图像、管理颜色和样式、管理 PDF 字体、将多个 PDF 文件合并为一个文件、将 PDF 文件拆分为多个文件、 根据页数、页面范围进行拆分、向 PDF 文件添加数字签名等等。
Aspose.PDF Cloud Ruby SDK 提供了简单直观的 REST API,适合各个级别的开发人员轻松使用。 SDK 提供了非常强大的功能,可使用 SDK 将 PDF 文件转换为其他支持的文件格式,例如 HTML、DOCX、JPEG、PNG、SVG、TIFF 和 XPS。 该库可用于处理各种类型的注释,例如直线注释、圆形注释、方形注释、下划线注释和波浪注释。 无论软件专业人员需要创建新的 PDF 文档还是编辑、操作或转换现有的 PDF 文件,Aspose.PDF Cloud Ruby SDK 都是他们下一个项目的绝佳选择。
Aspose.PDF Cloud Ruby SDK 入门
安装 Aspose.PDF for Python 的推荐方法是使用 RubyGems。 为了顺利安装,请使用以下命令。
使用 RubyGems 安装 Aspose.PDF Cloud Ruby SDK
gem install ./aspose_pdf_cloud-23.2.0.gem
您可以直接从Aspose.PDF产品页面下载该库
通过 Ruby API 创建 PDF 文件
Aspose.PDF Cloud Ruby SDK 是一个功能强大的 PDF 文档处理 API,允许软件开发人员仅用几行 Python 代码从头开始创建新的 PDF 文档。 该库还提供了其他几个用于 PDF 文档操作的功能,例如打开现有的 PDF 文件、向现有的 PDF 文件添加页面、从其他页面插入文本、从流中加载 PDF 文件、向 PDF 添加图像、将大型 PDF 文件拆分为较小的 PDF 文件。 一、将多个 PDF 文件合并为一个文件、从 PDF 中删除不需要的页面、打印 PDF 文件等等。
通过 Ruby API 从 HTML 创建 PDF 文件
class Document
include AsposePDFCloud
include AsposeStorageCloud
def initialize
# Get App key and App SID from https://cloud.aspose.com
AsposeApp.app_key_and_sid("APP_KEY", "APP_SID")
@pdf_api = PdfApi.new
end
def upload_file(file_name)
@storage_api = StorageApi.new
response = @storage_api.put_create(file_name, File.open("../../../data/" << file_name,"r") { |io| io.read } )
end
def create_pdf_from_html
file_name = "newPDFFromHTML.pdf"
template_file = "sample.html"
upload_file(template_file)
response = @pdf_api.put_create_document(file_name, {template_file: template_file, template_type: "html"})
end
end
document = Document.new()
puts document.create_pdf_from_html
通过 Ruby API 拆分和合并 PDF 文件
Aspose.PDF Cloud Ruby SDK 包含一些强大的功能,用于在 Ruby 应用程序中处理 PDF 文档。 Ruby 库允许软件开发人员在云中只需几行 Ruby 代码即可合并或拆分 PDF 文档。 它支持合并多个 PDF 文档、拆分大型 PDF 文件、签署 PDF 文档、附加 PDF 文档等功能。 以下示例展示了软件开发人员如何在云端合并多个 PDF 文件。
如何通过 Ruby REST API 合并多个 PDF 文件
PdfApi pdfApi = new PdfApi("API_KEY", "APP_SID");
String fileName = "sample-merged.pdf";
String storage = "";
String folder = "";
MergeDocuments body = new MergeDocuments();
body.List = new System.Collections.Generic.List { "sample.pdf", "input.pdf" };
try
{
// Upload source file to aspose cloud storage
pdfApi.UploadFile("sample.pdf", System.IO.File.ReadAllBytes(Common.GetDataDir() + "sample.pdf"));
pdfApi.UploadFile("input.pdf", System.IO.File.ReadAllBytes(Common.GetDataDir() + "input.pdf"));
// Invoke Aspose.PDF Cloud SDK API to merge pdf files
DocumentResponse apiResponse = pdfApi.PutMergeDocuments(fileName, storage, folder, body);
if (apiResponse != null && apiResponse.Status.Equals("OK"))
{
Console.WriteLine("Merge Multiple PDF Files, Done!");
Console.ReadKey();
}
}
catch (Exception ex)
{
System.Diagnostics.Debug.WriteLine("error:" + ex.Message + "\n" + ex.StackTrace);
}
通过 Ruby API 添加和管理 PDF 页面
Aspose.PDF Cloud Ruby SDK 提供了非常有用的功能,可以使用 Ruby API 处理 PDF 页面。 该库包含用于处理 PDF 页面的非常强大的功能,例如向 PDF 文档添加新页面、计算 PDF 页面并获取值、检索有关特定 PDF 页面的信息、获取 PDF 文档字数统计、从 PDF 中删除不需要的页面 文档、更改 PDF 文件内页面的位置、签署 PDF 页面、将 PDF 页面转换为图像格式等等。 以下示例演示了软件开发人员如何通过 Ruby 命令将 PDF 页面导出为 PNG 图像格式。
如何通过 Ruby 从 PDF 中删除页面?
require 'aspose_pdf_cloud'
class Page
include AsposePDFCloud
include AsposeStorageCloud
def initialize
# Get App key and App SID from https://cloud.aspose.com
AsposeApp.app_key_and_sid("APP_KEY", "APP_SID")
@pdf_api = PdfApi.new
end
def upload_file(file_name)
@storage_api = StorageApi.new
response = @storage_api.put_create(file_name, File.open("../../../data/" << file_name,"r") { |io| io.read } )
end
# Delete document page by its number.
def delete_page
file_name = "sample-input.pdf"
upload_file(file_name)
page_number = 1
response = @pdf_api.delete_page(file_name, page_number)
end
end
page = Page.new()
puts page.delete_page
通过 Ruby 管理 PDF 页眉/页脚和书签
页眉和页脚是 PDF 文档中非常重要的部分,它使用户能够放置有关文档的重要信息,并方便读者浏览文档。 大多数情况下,它通过包含他们希望出现在文档每一页上的材料来使开发人员的工作变得轻松。 Aspose.PDF Cloud Ruby SDK 完全支持向 PDF 文档添加页眉和页脚。 该库允许用户使用 Ruby 代码将文本、图像和其他 PDF 文件插入页眉和页脚。 软件开发人员还可以轻松添加新书签、更新书签、从 PDF 文件获取所有或特定书签等。
如何通过Ruby API从PDF文件中获取特定书签?
class Bookmark
include AsposePDFCloud
include AsposeStorageCloud
def initialize
# Get App key and App SID from https://cloud.aspose.com
AsposeApp.app_key_and_sid("APP_KEY", "APP_SID")
@pdf_api = PdfApi.new
end
def upload_file(file_name)
@storage_api = StorageApi.new
response = @storage_api.put_create(file_name, File.open("../../../data/" << file_name,"r") { |io| io.read } )
end
# Read document bookmarks.
def read_document_bookmarks
file_name = "Sample-Bookmark.pdf"
upload_file(file_name)
response = @pdf_api.get_document_bookmarks(file_name)
end
end
bookmark = Bookmark.new()
puts bookmark.read_document_bookmarks