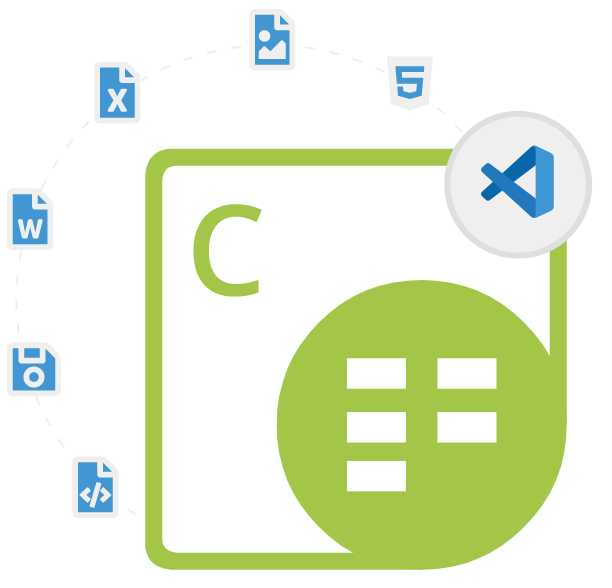
Aspose.Cells for .NET
用于创建和转换 Excel 电子表格的 C# .NET API
高级电子表格文档处理 API,无需使用 Microsoft PowerPoint 或其他第三方软件即可创建、修改、转换和呈现电子表格文件。
Aspose.Cells for .NET 是一个先进且功能丰富的库,使软件程序员能够创建 无需在计算机上安装 Microsoft Office 或 Excel 即可管理 Excel 电子表格。 该库支持您的企业每天使用的各种流行电子表格(XLS、XLSX、XLSM、XLSB、XLTX、XLTM、CSV、SpreadsheetML、ODS)文件格式。 除此之外,该库还支持将 Excel 电子表格导出为 PDF、DOCX、PPTX、JSON、XPS、HTML、MHTML、JSON、纯文本以及流行的图像格式,包括 TIFF、JPG、PNG、BMP 和 SVG。
Aspose.Cells for .NET 包含许多用于在 .NET 应用程序内处理电子表格文档创建及其管理的功能,例如向现有电子表格文件添加新工作簿、添加现有工作表的副本、添加图像和图表 、设置图表的渐变背景、创建注释、自动过滤器和分页符、使用 Excel 公式和计算、创建数据透视表、添加新工作簿、合并现有工作簿、导入图像和图表、从设计器电子表格导入公式,以及 还有很多。
Aspose.Cells for .NET 提供了广泛的附加功能,包括创建和操作图表、数据透视表和命名范围的能力,以及对数据验证、数据保护和条件格式化的支持。 该库可与任何类型的应用程序一起使用,无论是 ASP.NET Web 应用程序还是 Windows 桌面应用程序。 凭借其广泛的功能、对多种文件格式的支持以及丰富的文档,Aspose.Cells 是任何希望在 .NET 应用程序中使用 Excel 文件的开发人员的绝佳选择。
开始使用 Aspose.Cells for .NET
安装 Aspose.Cells for .NET 的推荐方法是使用 NuGet。 为了顺利安装,请使用以下命令。
通过 NuGet 安装 Aspose.Cells for .NET
NuGet\Install-Package Aspose.Cells -Version 23.1.1
您也可以直接从Aspose 产品发布页面下载。通过 C#.NET API 创建 Excel 电子表格
Aspose.Cells for .NET 完全支持多种文件格式之间的转换。 它允许软件开发人员以一种文件格式加载 Excel 电子表格,并将其以多种其他受支持的文件格式保存在其 .NET 应用程序中。 该库允许将 Excel 电子表格转换为 PDF、HTML、PowerPoint、XPS、HTML、MHTML、JSON、纯文本和流行的图像格式,包括 TIFF、JPG、PNG、BMP 和 SVG。 该库还允许将 Excel 工作簿转换为 Ods、Sxc 和 Fods (OpenOffice / LibreOffice calc)。
通过 .NET API 创建新工作簿
string dataDir = RunExamples.GetDataDir(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
try
{
// Create a License object
License license = new License();
// Set the license of Aspose.Cells to avoid the evaluation limitations
license.SetLicense(dataDir + "Aspose.Cells.lic");
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
// Instantiate a Workbook object that represents Excel file.
Workbook wb = new Workbook();
// When you create a new workbook, a default "Sheet1" is added to the workbook.
Worksheet sheet = wb.Worksheets[0];
// Access the "A1" cell in the sheet.
Cell cell = sheet.Cells["A1"];
// Input the "Hello World!" text into the "A1" cell
cell.PutValue("Hello World!");
// Save the Excel file.
wb.Save(dataDir + "MyBook_out.xlsx");
通过 C# .NET API 保护 Excel 电子表格
Aspose.Cells for .NET 使软件开发人员能够保护或取消保护其 .NET 应用程序内的电子表格文档。 该库包含了几个用于保护电子表格文件及其内部数据的重要功能,例如通过应用密码防止其他人访问 Excel 文件中的数据、保护和取消保护工作簿或工作表、添加数字签名等等。该库还支持 防止查看隐藏的工作表、添加、移动、删除或隐藏工作表以及重命名工作表。
通过 .NET API 密码保护或取消保护共享工作簿
Workbook wb = new Workbook();
//Protect the Shared Workbook with Password
wb.ProtectSharedWorkbook("1234");
//Uncomment this line to Unprotect the Shared Workbook
//wb.UnprotectSharedWorkbook("1234");
//Save the output Excel file
wb.Save("outputProtectSharedWorkbook.xlsx");
通过 C# 支持 Excel 公式计算
Aspose.Cells for .NET 包含使用 Excel 公式和使用 C#.NET 命令计算结果的功能。 该库提供了一套全面的函数来处理 Excel 公式,使创建和操作公式以及评估和重新计算变得容易。 支持直接计算公式、重复计算公式、Excel 2016 MINIFS和MAXIFS函数计算、IFNA函数计算、数据表数组公式计算等。
通过 C# API 设置命名范围的简单公式
string dataDir = RunExamples.GetDataDir(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
// Create an instance of Workbook
Workbook book = new Workbook();
// Get the WorksheetCollection
WorksheetCollection worksheets = book.Worksheets;
// Add a new Named Range with name "NewNamedRange"
int index = worksheets.Names.Add("NewNamedRange");
// Access the newly created Named Range
Name name = worksheets.Names[index];
// Set RefersTo property of the Named Range to a formula. Formula references another cell in the same worksheet
name.RefersTo = "=Sheet1!$A$3";
// Set the formula in the cell A1 to the newly created Named Range
worksheets[0].Cells["A1"].Formula = "NewNamedRange";
// Insert the value in cell A3 which is being referenced in the Named Range
worksheets[0].Cells["A3"].PutValue("This is the value of A3");
// Calculate formulas
book.CalculateFormula();
// Save the result in XLSX format
book.Save(dataDir + "output_out.xlsx");
通过 C# API 合并多个 Excel 文件和工作簿
通常需要将各种 Excel 文件或工作簿合并到一个电子表格文件中。 Aspose.Cells for .NET 使软件开发人员可以轻松地使用几行 .NET 代码将包含图像、图表、文本和其他数据的多个工作簿合并到一个工作簿中。 该库还支持轻松地将多个工作表合并为一个工作表。
通过 .NET API 合并多个工作簿
string dataDir = RunExamples.GetDataDir(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
// Define the first source
// Open the first excel file.
Workbook SourceBook1 = new Workbook(dataDir+ "SampleChart.xlsx");
// Define the second source book.
// Open the second excel file.
Workbook SourceBook2 = new Workbook(dataDir+ "SampleImage.xlsx");
// Combining the two workbooks
SourceBook1.Combine(SourceBook2);
dataDir = dataDir + "Combined.out.xlsx";
// Save the target book file.
SourceBook1.Save(dataDir);