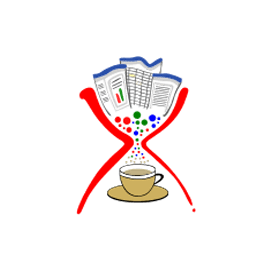
Apache POI XWPF
用于 Word OOXML 文档的 Java API
在 Java 应用程序中创建、读取、编辑和转换 Microsoft Word DOCX 文件的开源解决方案。
Apache POI XWPF 入门
首先,您需要在系统上安装 Java 开发工具包 (JDK)。如果您已经拥有它,请继续访问 Apache POI 的 下载 页面以获取存档中的最新稳定版本。将 ZIP 文件的内容提取到可以将所需库链接到 Java 程序的任何目录中。就这些!
在基于 Maven 的 Java 项目中引用 Apache POI 更加简单。您只需在 pom.xml 中添加以下依赖项,并让您的 IDE 获取和引用 Apache POI Jar 文件。
Apache POI Maven 依赖
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.0</version>
</dependency>
使用 Java API 生成和编辑 Word 文档
Apache POI XWPF 使软件程序员能够以 DOCX 文件格式创建新的 Word 文档。开发人员还可以加载现有的 Microsoft Word DOCX 文件以根据他们的应用程序需要对其进行编辑。它允许您添加新段落、插入文本、应用文本对齐和边框、更改文本样式等。
从头开始生成 DOCX 文件
// initialize a blank document
XWPFDocument document = new XWPFDocument();
// create a new file
FileOutputStream out = new FileOutputStream(new File("document.docx"));
// create a new paragraph paragraph
XWPFParagraph paragraph = document.createParagraph();
XWPFRun run = paragraph.createRun();
run.setText("File Format Developer Guide - " +
"Learn about computer files that you come across in " +
"your daily work at: www.fileformat.com ");
document.write(out);
out.close();
将段落、图像和表格添加到 Word 文档
Apache POI XWPF 允许开发人员将段落和图像添加到 Word 文档中。 API 还提供了将表格添加到 DOCX 文档的功能,同时可以使用用户定义的数据创建简单的嵌套表格。
使用表格创建新的 DOCX 文件
// initialize a blank document
XWPFDocument document = new XWPFDocument();
// create a new file
FileOutputStream out = new FileOutputStream(new File("table.docx"));
// create a new table
XWPFTable table = document.createTable();
// create first row
XWPFTableRow tableRowOne = table.getRow(0);
tableRowOne.getCell(0).setText("Serial No");
tableRowOne.addNewTableCell().setText("Products");
tableRowOne.addNewTableCell().setText("Formats");
// create second row
XWPFTableRow tableRowTwo = table.createRow();
tableRowTwo.getCell(0).setText("1");
tableRowTwo.getCell(1).setText("Apache POI XWPF");
tableRowTwo.getCell(2).setText("DOCX, HTML, FO, TXT, PDF");
// create third row
XWPFTableRow tableRowThree = table.createRow();
tableRowThree.getCell(0).setText("2");
tableRowThree.getCell(1).setText("Apache POI HWPF");
tableRowThree.getCell(2).setText("DOC, HTML, FO, TXT");
document.write(out);
out.close();
从 Word OOXML 文档中提取文本
Apache POI XWPF 提供了专门的类,只需几行代码即可从 Microsoft Word DOCX 文档中提取数据。同样,它还可以从Word文件中提取标题、脚注、表格数据等。
从 Word 文件中提取文本
// load DOCX file
FileInputStream fis = new FileInputStream("document.docx");
// open file
XWPFDocument file = new XWPFDocument(OPCPackage.open(fis));
// read text
XWPFWordExtractor ext = new XWPFWordExtractor(file);
// display text
System.out.println(ext.getText());
将自定义页眉和页脚添加到 DOCX 文档
页眉和页脚是 Word 文档的重要组成部分,因为它们通常包含额外的信息,例如日期、页码、作者姓名和脚注,这有助于使较长的文档井井有条并且更易于阅读。 Apache POI XWPF 允许 Java 开发人员向 Word 文档添加自定义页眉和页脚。
在 Word DOCX 文件中管理自定义页眉和页脚
public class HeaderFooterTable {
public static void main(String[] args) throws IOException {
try (XWPFDocument doc = new XWPFDocument()) {
// Create a header with a 1 row, 3 column table
XWPFHeader hdr = doc.createHeader(HeaderFooterType.DEFAULT);
XWPFTable tbl = hdr.createTable(1, 3);
// Set the padding around text in the cells to 1/10th of an inch
int pad = (int) (.1 * 1440);
tbl.setCellMargins(pad, pad, pad, pad);
// Set table width to 6.5 inches in 1440ths of a point
tbl.setWidth((int) (6.5 * 1440));
CTTbl ctTbl = tbl.getCTTbl();
CTTblPr ctTblPr = ctTbl.addNewTblPr();
CTTblLayoutType layoutType = ctTblPr.addNewTblLayout();
layoutType.setType(STTblLayoutType.FIXED);
BigInteger w = new BigInteger("3120");
CTTblGrid grid = ctTbl.addNewTblGrid();
for (int i = 0; i < 3; i++) {
CTTblGridCol gridCol = grid.addNewGridCol();
gridCol.setW(w);
}
// Add paragraphs to the cells
XWPFTableRow row = tbl.getRow(0);
XWPFTableCell cell = row.getCell(0);
XWPFParagraph p = cell.getParagraphArray(0);
XWPFRun r = p.createRun();
r.setText("header left cell");
cell = row.getCell(1);
p = cell.getParagraphArray(0);
r = p.createRun();
r.setText("header center cell");
cell = row.getCell(2);
p = cell.getParagraphArray(0);
r = p.createRun();
r.setText("header right cell");
// Create a footer with a Paragraph
XWPFFooter ftr = doc.createFooter(HeaderFooterType.DEFAULT);
p = ftr.createParagraph();
r = p.createRun();
r.setText("footer text");
try (OutputStream os = new FileOutputStream(new File("headertable.docx"))) {
doc.write(os);
}
}
}
}